In this blogpost series we will zoom in on the use of DataTables in a Django application with HTML frontend. In this post we will focus on formatting our dates (dd-MM-YYYY). The full code of the tutorial can be found on my Github.
This tutorial follows the examples from our previous posts. You do not need these to follow along. Previous posts:
- Python Django DataTables: #1 Creating a basic jQuery DataTable
- Python Django DataTables: #2 Jquery DataTable Display Data with JSON Response
In the previous posts we finished with the table below. As you can see, the dates are not in a readible format. During this tutorial we will use Moments to make sure that our data can be displayed in different formats.
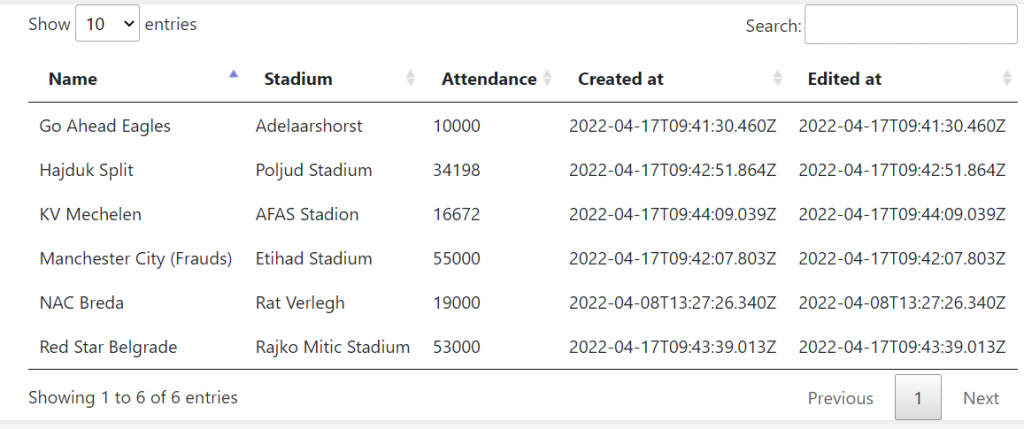
To correct the format we follow the following steps:
- Adding the scripts to our HTML file
- Add moment.js code to the DataTable
- Changing our column date format
Adding the scripts
In order to format your dates and use them for filtering we need to add two scripts which are provided on the datatables website. The first script enables us to use moment.js, the second script enables sorting. Add the scripts below on top of your HTML file.
base.html
<script src="//cdnjs.cloudflare.com/ajax/libs/moment.js/2.8.4/moment.min.js"></script>
<script src="//cdn.datatables.net/plug-ins/1.10.21/sorting/datetime-moment.js"></script>
Add moment.js code to DataTable
We now need to add the moment.js code to our DataTable. Add the green code below to your datatable script. This line will make sure that we can format our columns.
table3.html
<script>
$(document).ready(function() {
$.fn.dataTable.moment('DD-MM-YYYY');
var table = $('#ourtable2').DataTable({
"ajax": {
"processing": true,
"url": "{% url 'footballclubs' %}",
"dataSrc": "",
},
"columns": [
{ "data": "name"},
{ "data": "stadium"},
{ "data": "attendance"},
{ "data": "created_at"},
{ "data": "edited_at"},
],
});
});
</script>
Changing our column date format
So far, nothing has changed to the format of our dates. We need to make a change to our columns in order to show the correct date format. In our columns we add a render method with a function that formats the dates in the DD-MM-YYYY format. If the data is null, no formatting will be applied.
table3.html
$(document).ready(function() {
$.fn.dataTable.moment('DD-MM-YYYY');
var table = $('#ourtable2').DataTable({
"ajax": {
"processing": true,
"url": "{% url 'footballclubs' %}",
"dataSrc": "",
},
"columns": [
{ "data": "name"},
{ "data": "stadium"},
{ "data": "attendance"},
{'data': "created_at",
"render": function (data) { if (data === null) return "";
return window.moment(data).format('DD-MM-YYYY')}
},
{'data': "edited_at",
"render": function (data) { if (data === null) return "";
return window.moment(data).format('DD-MM-YYYY')}
},
],
});
});
If we now start our server, we will see that our dates are formatted. Sorting the dates will also work correctly.
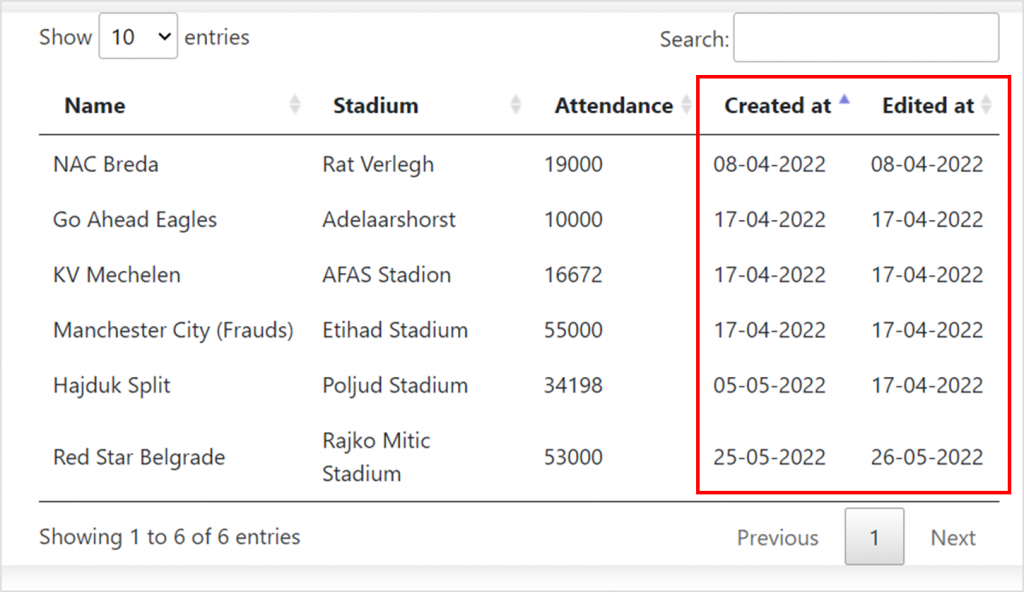
Change the format of your dates
You can change the format of your dates by changing the moment code on the top of the DataTable and the render format in the columns.
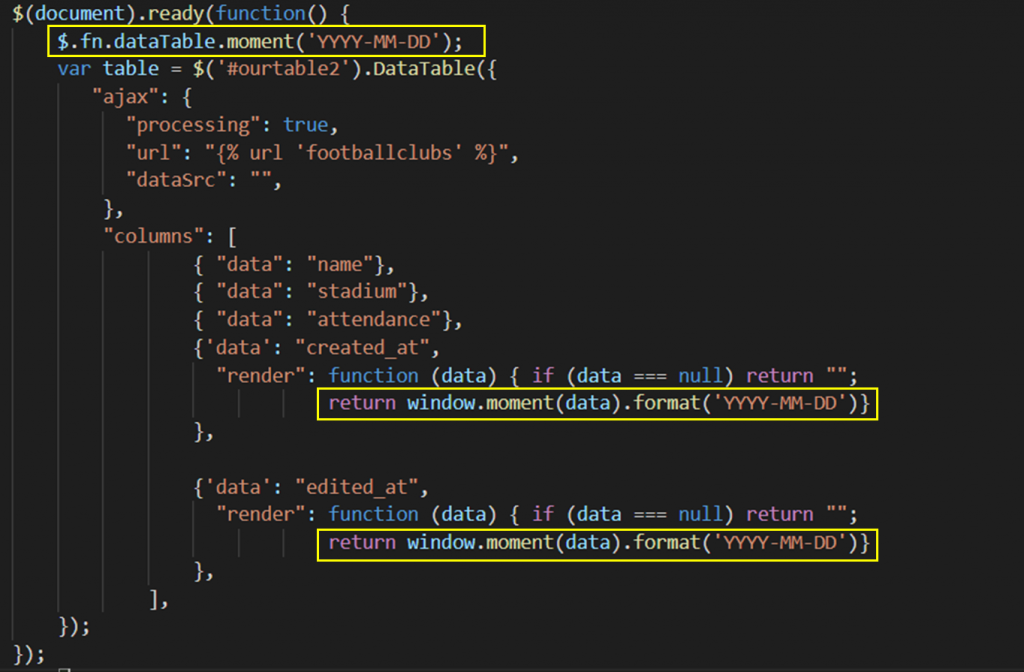
Our dates are formatted!
We are all set! Our dates can now be changed and sorted in a correct manner. In the next blogpost we will focus on styling our table. The full code of the project can be found on my Github.